Scenario: Batch PDF to Word Conversion Without Licensed Software or Cloud Upload.
You have multiple PDF files that need to be converted to Word documents. However, you want to avoid using costly licensed software like Adobe Acrobat Pro and are concerned about uploading sensitive files to free online converters due to privacy risks.
Instead, you can use a free and secure offline solution, like a Python script with open-source libraries, to automate the conversion process on your local machine. This ensures your data remains private while saving you money on software licenses.
Step 1: Install Python
You can download it from python.org. Make sure to check the box “Add Python to PATH” during installation.
Step 2: Set Up Your VS Code Environment
- Open VS Code.
- Create a new folder for this project (e.g, PDF-WORD).
- Open this folder in VS Code (File > Open Folder).
Step 3: Install Required Python Libraries
Open your command terminal and run the following command to install pdf2docx libraries.
pip install pdf2docx
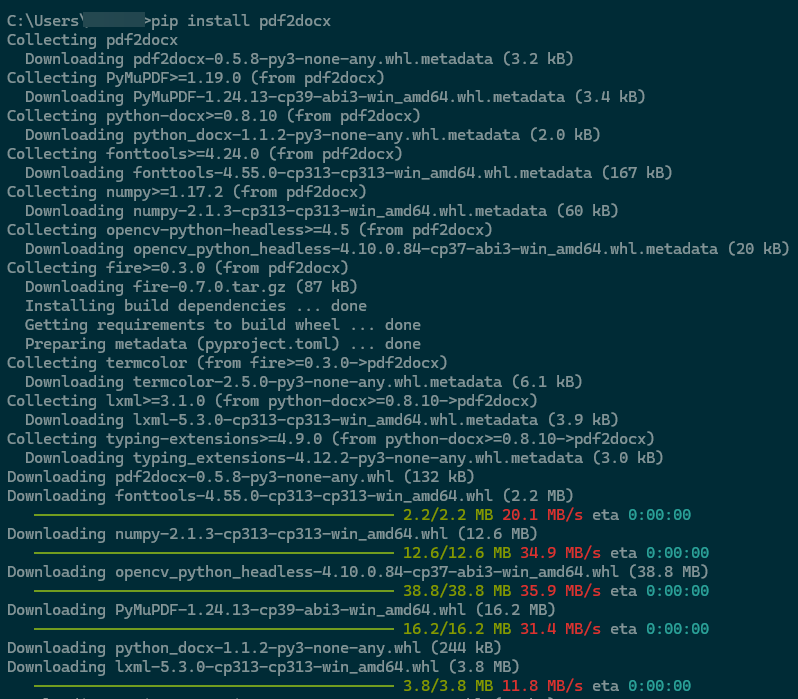
Step 4: Arrange Your input/output Folders
Create two subfolders within your project directory:
pdf_files
(to store your source PDF files)word_files
(to save the converted Word documents)
Step 4: Write the Python Script
Create a new python file in VS Code and name it (e.g, convertpdf.py). copy and paste below code
import os
from pdf2docx import Converter
# Set the source and destination folder paths
source_folder = 'pdf_files'
destination_folder = 'word_files'
# Ensure the destination folder exists
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
# Function to convert PDF to Word
def convert_pdf_to_word(source_path, dest_path):
try:
print(f"Converting {source_path} to {dest_path}...")
cv = Converter(source_path)
cv.convert(dest_path)
cv.close()
print(f"Successfully converted: {source_path}")
except Exception as e:
print(f"Failed to convert {source_path}. Error: {e}")
# Iterate over all PDF files in the source folder
for filename in os.listdir(source_folder):
if filename.lower().endswith('.pdf'):
source_path = os.path.join(source_folder, filename)
dest_path = os.path.join(destination_folder, filename.replace('.pdf', '.docx'))
convert_pdf_to_word(source_path, dest_path)
print("All files have been processed.")
Step 6: Input Your PDF files
- place all your PDF files in the pdf_files folder
Step 7: Run the Python Script
- Open your convertpdf.py in VS Code.
- Run the script by pressing F5 or using the terminal.
python convertpdf.py
Step 8: Verify Your Output
- Converted Word documents should appear in the word_files folder.
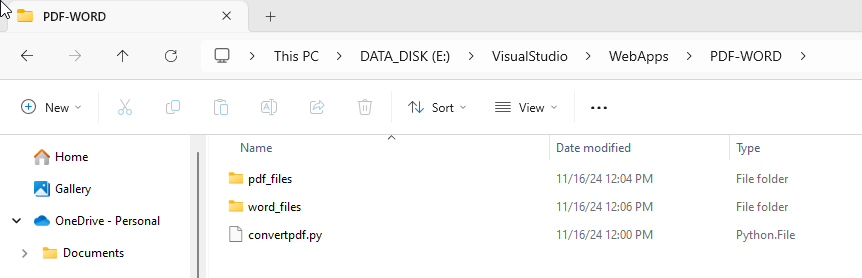
This idea is inspired by Mohammad Haneef. Kudos to him.